Newsgator Social Sites 2010 is a great social add-on for SharePoint 2010 with a lot of features (IMHO maybe too many) and an extensive API to manage programmatically the Activity Stream, the creation of Communities, the Followers of a Community… they have a great support community (https://engage.newsgator.com) where, if you are a partner, you can register yourself to ask the real experts and access special documentation.
In my current project I needed to create a SiteDefintion with several default Newsgator tabs. It seems really easy to go to the Setup page and create a new Blog or Discussion Board using the UI, but to be honest it was really hard to find any kind of documentation about how to do it programmatically (in fact it was impossible).
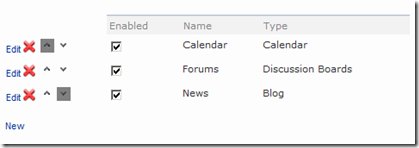
My idea was to provide a SPWebEventReceiver event handler and add in the WebProvisioned event the tabs creation (they have special names, special webparts inside…). Within the following code and guessing what was Newsgator internally doing to add the tabs in the UI (like creating a new “blog” or creating a new “calendar” list), I was able to add them.
///
/// A site was provisioned.
///
public override void WebProvisioned(SPWebEventProperties properties)
{
base.WebProvisioned(properties);
SPWeb web = properties.Web;
if (web.WebTemplate.Equals("mySiteDefinition", StringComparison.InvariantCultureIgnoreCase))
{
// Blog
SPWeb blog = web.Webs.Add("news", "News", "News description", 1033, "BLOG#0", false, false);
var tabs = new List();
tabs.Add(new CommunityTab()
{
Id = 0,
CapabilityType = CapabilityType.Overview,
Name = "Home",
Enabled = true,
DisplayType = ServerUI.OverviewTab,
TabIndex = 0
});
tabs.Add(new CommunityTab()
{
Id = 1,
CapabilityType = CapabilityType.List,
Name = "Calendar",
Enabled = true,
DisplayType = "Calendar",
TabIndex = 1,
TemplateType = SPListTemplateType.Events
});
tabs.Add(new CommunityTab()
{
Id = 2,
CapabilityType = CapabilityType.DiscussionBoards,
Name = "Forums",
Enabled = true,
DisplayType = ServerUI.CommunityTabType_DiscussionBoards,
TabIndex = 2,
});
tabs.Add(new CommunityTab()
{
Id = 3,
CapabilityType = CapabilityType.Web,
Name = "News",
Enabled = true,
TabIndex = 3,
DisplayType = ServerUI.CommunityTabType_Blog,
SubWebId = blog.ID
});
SocialGroupPrivacyLevel privacyLevel = SocialGroupPrivacyLevel.Private;
CommunitySetup newsgatorSetup = new CommunitySetup();
newsgatorSetup.SaveSetupConfiguration(web.Site, web, tabs, web.Title, true, privacyLevel, false);
web.Features.Add(CommunityGlobals.WebCommunityFeature, true); // Convert to community before add blog
web.Features.Add(CommunityGlobals.WebScopedSkinningFeature, true); // Skin for NG communities
web.Dispose();
}
}
Anyway, with this code I had 2 serious problems:
- the blog linked to the “News” tab didn’t have the top Newsgator navigation menu… it seemed like it was an isolated blog, and that was not what I wanted (maybe I needed a NG feature?)
- the discussions board didn’t show up… and the “boards.aspx” page that it’s deployed using the UI was simply not there… (maybe again I needed a NG feature?)
Because of that lack of documentation, I was “forced” to glimpse the DLLs using ILSpy (great application when you are in trouble) and I realized I needed to change the code a little bit:
- activate the NewsGator.Communities_NewsGator.Community.Context feature in the blog to enable the top navigation menu.
- activate the NewsGator.Communities.BoardsPage feature in my site, which deploys the boards.aspx page.
- add the LinkUrl property in the Discussions Boards page to “boards.aspx”
At the end, my successful code looked like:
///
/// A site was provisioned.
///
public override void WebProvisioned(SPWebEventProperties properties)
{
base.WebProvisioned(properties);
SPWeb web = properties.Web;
if (web.WebTemplate.Equals("mySiteDefinition", StringComparison.InvariantCultureIgnoreCase))
{
// Blog
SPWeb blog = web.Webs.Add("news", "News", "News description", 1033, "BLOG#0", false, false);
var tabs = new List();
tabs.Add(new CommunityTab()
{
Id = 0,
CapabilityType = CapabilityType.Overview,
Name = "Home",
Enabled = true,
DisplayType = ServerUI.OverviewTab,
TabIndex = 0
});
tabs.Add(new CommunityTab()
{
Id = 1,
CapabilityType = CapabilityType.List,
Name = "Calendar",
Enabled = true,
DisplayType = "Calendar",
TabIndex = 1,
TemplateType = SPListTemplateType.Events
});
tabs.Add(new CommunityTab()
{
Id = 2,
CapabilityType = CapabilityType.DiscussionBoards,
Name = "Forums",
Enabled = true,
DisplayType = ServerUI.CommunityTabType_DiscussionBoards,
TabIndex = 2,
LinkUrl = "boards.aspx"
});
tabs.Add(new CommunityTab()
{
Id = 3,
CapabilityType = CapabilityType.Web,
Name = "News",
Enabled = true,
TabIndex = 3,
DisplayType = ServerUI.CommunityTabType_Blog,
SubWebId = blog.ID
});
SocialGroupPrivacyLevel privacyLevel = SocialGroupPrivacyLevel.Private;
CommunitySetup newsgatorSetup = new CommunitySetup();
newsgatorSetup.SaveSetupConfiguration(web.Site, web, tabs, web.Title, true, privacyLevel, false);
blog.Features.Add(new Guid("b084760c-452e-419d-8639-babb5c0a4283"), true); // NewsGator.Communities_NewsGator.Community.Context feature
web.Features.Add(CommunityGlobals.WebCommunityFeature, true); // Convert to community before add blog
web.Features.Add(CommunityGlobals.WebScopedSkinningFeature, true); // Skin for NG communities
web.Features.Add(new Guid("62ab07ce-3f9f-441a-80ce-b14beae97dd4"), true); // NewsGator.Communities.BoardsPage feature
blog.Dispose();
web.Dispose();
}
}
And it added the wished tabs, working properly.

Hope this helps someone!